一、轮播的实现原理以及抽象出哪些函数(or接口)供使用?
- 轮播的实现原理
- 把需要展示的图片放到同一行。
- 创建一个可视化窗口,窗口之外的区域隐藏。
- 在第一张图片的前面克隆最后一个图片元素,在最后一个图片后面克隆第一个元素。
- 利用jQuery动画以及定位就能实现无限循环的轮播。
- 抽象出来的函数
- play(playPre()、playNext())
- setBullet()(点击下面的小横线或者图片的缩略图进行播放)
二、实现左右滚动无限循环轮播效果。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>无限轮播</title>
<style>
*{
margin: 0;
padding: 0;
}
li{
list-style: none;
}
a{
text-decoration: none;
}
.clearfix:after{
content: '';
display: block;
clear: both;
}
.rotation{
position: relative;
width: 600px;
height: 350px;
overflow: hidden;
margin: 30px auto;
text-align: center;
}
.rotation .img-ct{
position: absolute;
/*width: 2400px;*/
/*宽度用js动态来写,不写死。图片排成一行*/
height: 350px;
}
.rotation .img-ct>li{
float: left;
}
.rotation img{
width: 600px;
height: 350px;
}
.rotation .btn{
position: absolute;
top: 50%;
transform: translate(-50%);
border-radius: 50%;
display: inline-block;
width: 40px;
height: 40px;
color: #fff;
font-size: 25px;
line-height: 40px;
background: #4E443C;
opacity: 0.7;
}
.rotation .btn:hover{
opacity: .4;
}
.rotation .btn-pre{
left: 40px;
}
.rotation .btn-next{
right: 0px;
}
.rotation .slide{
position: absolute;
left: 50%;
transform: translate(-50%);
bottom: 10px;
text-align: center;
}
.rotation .slide>li{
height: 5px;
width: 20px;
background: #fff;
display: inline-block;
border-radius: 5px;
cursor: pointer;
}
.rotation .slide .active{
background: #333;
opacity: .7;
}
</style>
</head>
<body>
<div class="rotation">
<ul class="img-ct clearfix">
<li key="0"><a href="#"></a></li>
<li key="1"><a href="#"></a></li>
<li key="2"><a href="#"></a></li>
<li key="3"><a href="#"></a></li>
</ul>
<a class="btn btn-pre" href="#"><</a>
<a class="btn btn-next" href="#">></a>
<ul class="slide">
<li class="active"></li>
<li></li>
<li></li>
<li></li>
</ul>
</div>
<script src="https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
var $imgCt = $('.rotation .img-ct'),
$slide = $('.slide'),
$btnPre = $('.rotation .btn-pre'),
$btnNext = $('.rotation .btn-next');
var imgLen = $imgCt.children().length; // 4
var key = 0;
var isAnimate = false;
var $firstImg = $imgCt.find('li').first(),
$lastImg = $imgCt.find('li').last();
$imgCt.append($firstImg.clone());
$imgCt.prepend($lastImg.clone());
$imgCt.width((imgLen+2)*$firstImg.width());
$imgCt.css('left', 0-$firstImg.width());
// 点击左右图标轮播
$btnPre.on('click', function(e) {
e.preventDefault();
playPre();
// console.log(key);
})
$btnNext.on('click', function(e) {
e.preventDefault();
playNext();
// console.log(key);
})
// 点击下面的小横线轮播
$slide.find('li').on('click', function(e) {
e.preventDefault();
var clickIndex = $(this).index();
if(clickIndex < key) {
playPre(clickIndex);
}else if(clickIndex > key){
playNext(clickIndex);
}else{
return;
}
})
function playPre(index) {
if(isAnimate) {
return;
}
isAnimate = true;
switch (typeof index)
{
case 'undefined':
$imgCt.animate({
left: '+=600px', // 别写成'+= 600px'
}, function() {
key--;
if(key < 0) {
$imgCt.css('left', 0-(imgLen*$firstImg.width()));
key = imgLen -1;
}
setSlide();
isAnimate = false;
})
break;
case 'number':
clickSlide(index);
break;
}
}
function playNext(index) {
if(isAnimate) {
return;
}
isAnimate = true;
switch (typeof index)
{
case 'undefined':
$imgCt.animate({
left: '-=600px',
}, function() {
key ++;
if(key == imgLen) {
$imgCt.css('left', '-600px');
key = 0;
}
setSlide();
isAnimate = false;
})
break;
case 'number':
clickSlide(index);
break;
}
// setSlide(); 动画是异步的,放在这里执行的时候,key还没有改变
// isAnimate = false; 动画是异步的!!!!!等动画完成之后再置为false
}
function clickSlide(index) {
var leftOffset= -(index+1)*$firstImg.width();
$imgCt.animate({
'left': leftOffset,
}, function() {
key = index;
setSlide();
isAnimate = false;
})
}
function setSlide() {
$('.rotation .slide').find('li').removeClass('active').eq(key).addClass('active');
console.log('key:' + key);
}
// 代码写的不是很美,但是基本功能都实现了,有待改善。
</script>
</body>
</html>
三、实现一个渐变轮播效果效果范例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>渐变效果的轮播</title>
<style>
*{
margin: 0;
padding: 0;
}
li{
list-style: none;
}
a{
text-decoration: none;
}
.clearfix:after{
content: '';
display: block;
clear: both;
}
.carousel{
position: relative;
width: 890px;
height: 500px;
overflow: hidden;
margin: 30px auto 0 auto;
}
.carousel .img-ct{
position: relative;
height: 500px;
}
.carousel .img-ct>li{
position: absolute;
display: none;
}
.carousel .img-ct img{
width: 890px;
}
.carousel .btn{
position: absolute;
top: 50%;
transform: translate(-50%);
border-radius: 50%;
display: inline-block;
width: 40px;
height: 40px;
color: #fff;
font-size: 25px;
line-height: 40px;
background: #4E443C;
opacity: 0.7;
text-align: center;
}
.carousel .btn:hover{
opacity: .4;
}
.carousel .btn-before{
left: 40px;
}
.carousel .btn-after{
right: 0px;
}
.thubms{
margin: 0 auto 30px auto;
width: 870px;
height: 80px;
}
.thubms .sample{
height: 80px;
padding: 10px 0;
margin: 0 -10px;
background: #000;
/*opacity: .4;*/
}
.thubms .sample li{
width: 100px;
height: 80px;
float: left;
margin-left: 10px;
opacity: 0.4;
cursor: pointer;
}
.thubms .sample li:hover{
opacity: 1;
}
.thubms .sample li img{
width: 100px;
height: 80px;
/*opacity: 0.4;*/
}
.thubms .sample .selected{
opacity: 1 !important;
/*background: '';*/
}
</style>
</head>
<body>
<div class="carousel clearfix">
<ul class="img-ct clearfix">
<li key="0"><a href="#"></a></li>
<li key="1"><a href="#"></a></li>
<li key="2"><a href="#"></a></li>
<li key="3"><a href="#">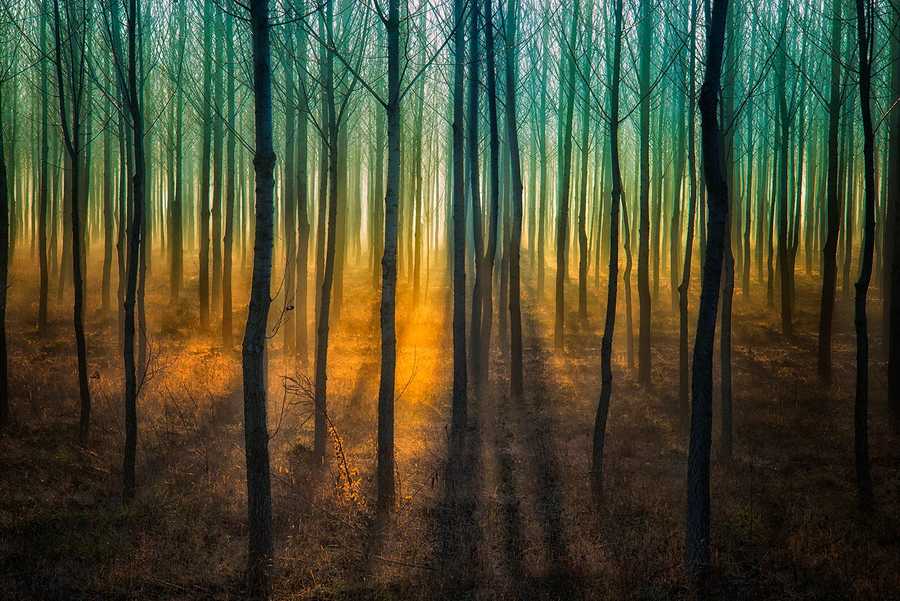</a></li>
<li key="4"><a href="#">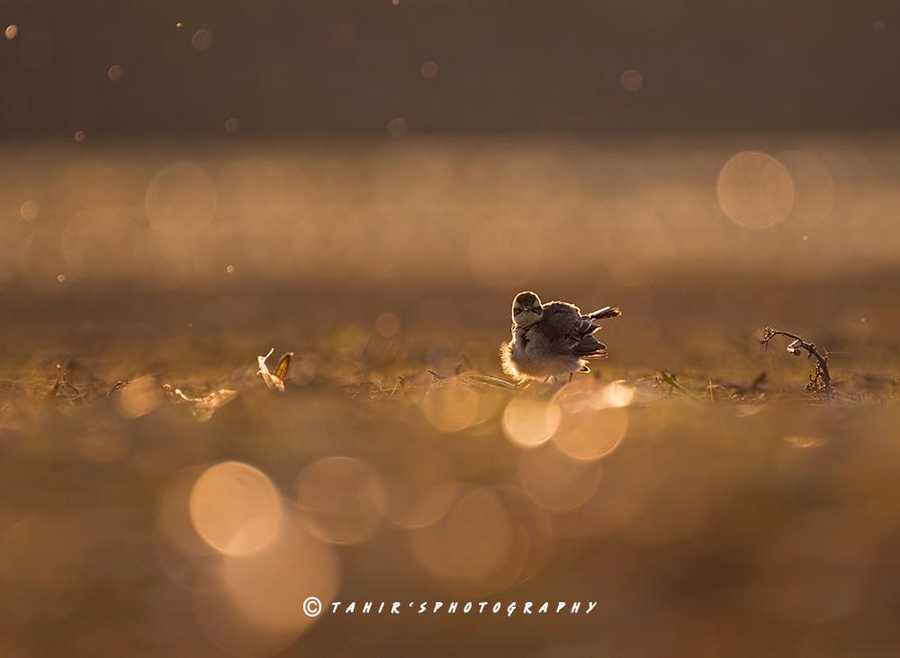</a></li>
<li key="5"><a href="#"></a></li>
<li key="6"><a href="#">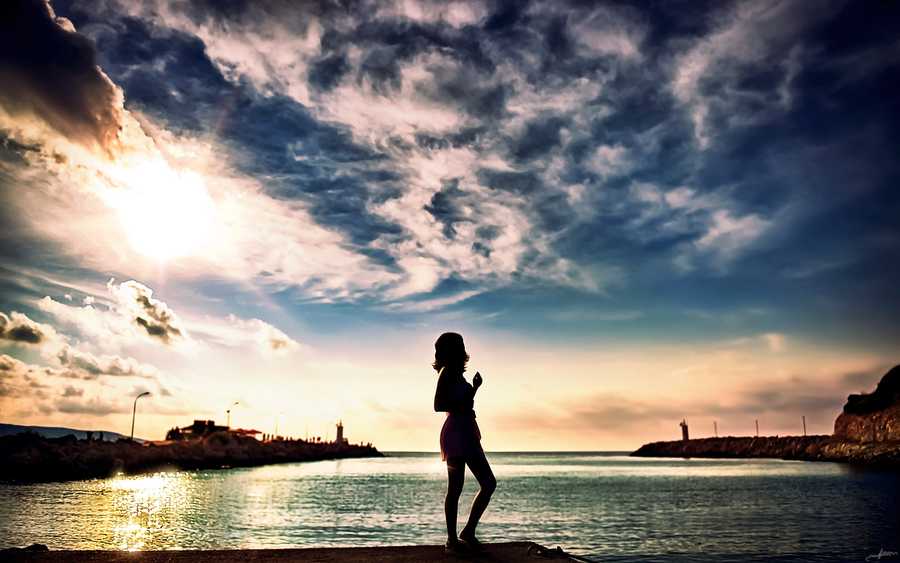</a></li>
<li key="7"><a href="#">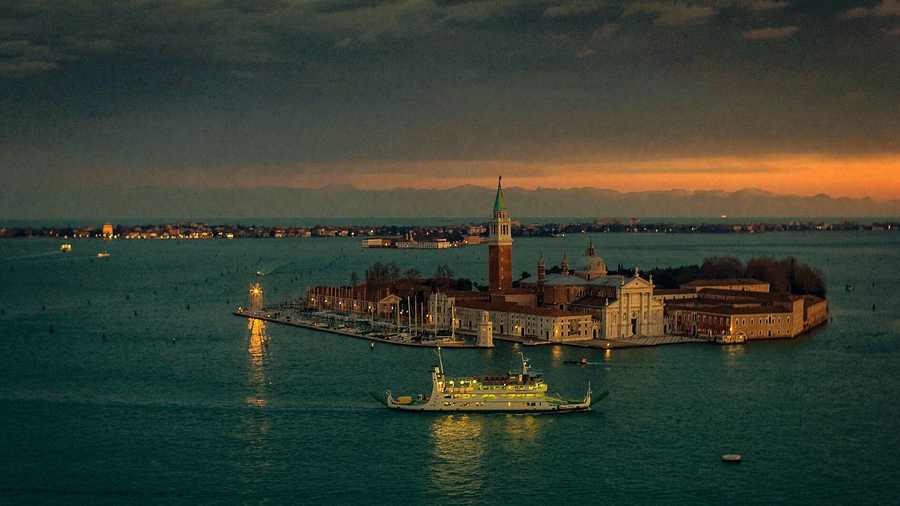</a></li>
</ul>
<a class="btn btn-before" href="#"><</a>
<a class="btn btn-after" href="#">></a>
</div>
<div class="thubms">
<ul class="sample clearfix">
<li class="selected"></li>
<li></li>
<li></li>
<li>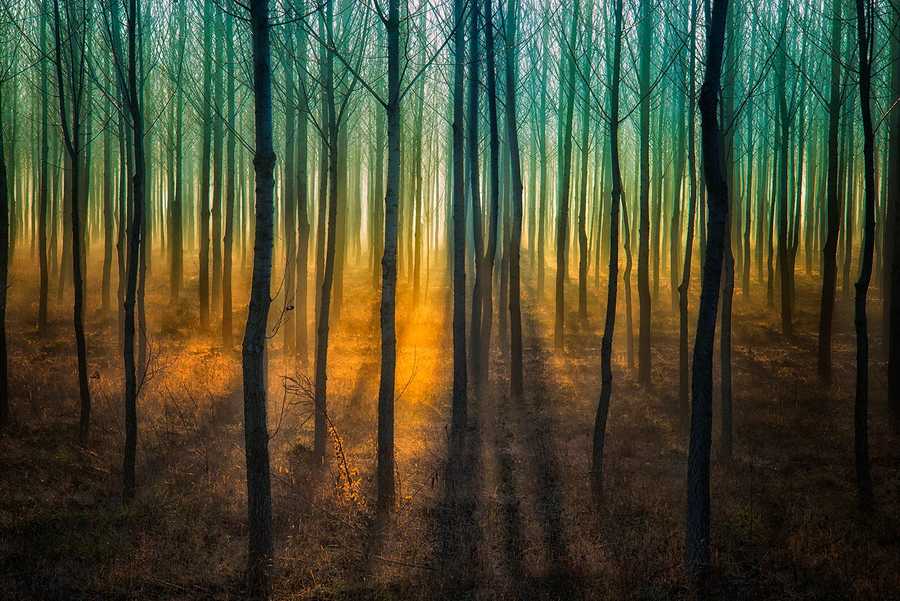</li>
<li>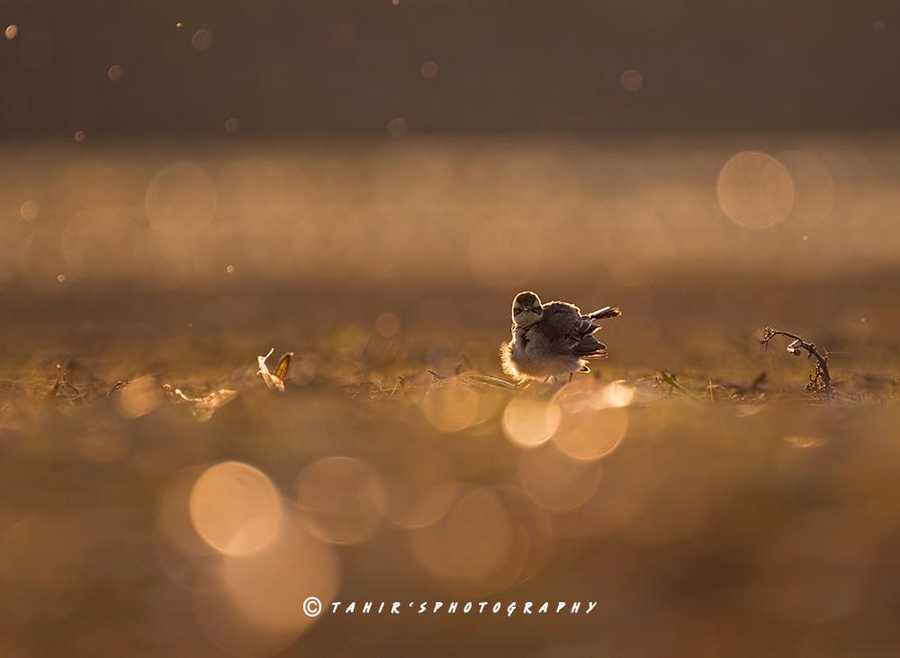</li>
<li></li>
<li>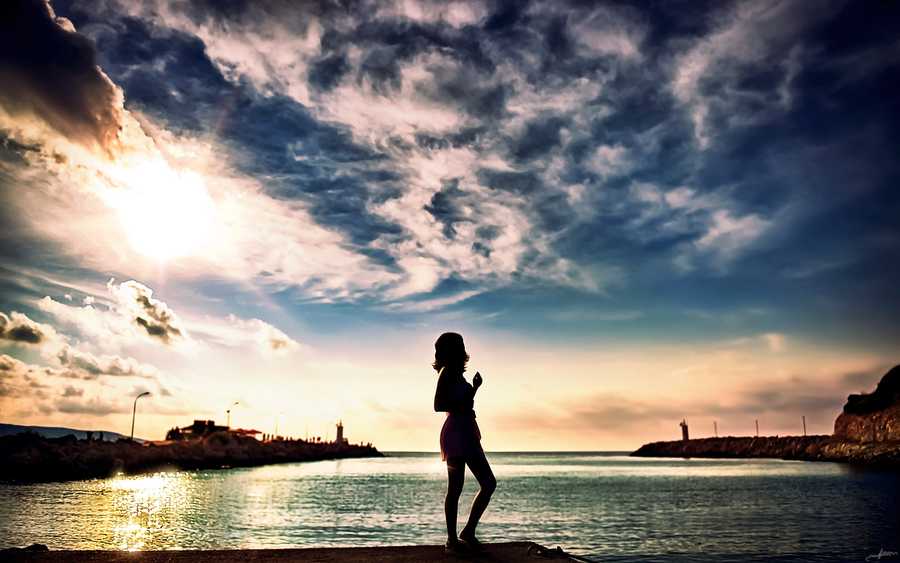</li>
<li>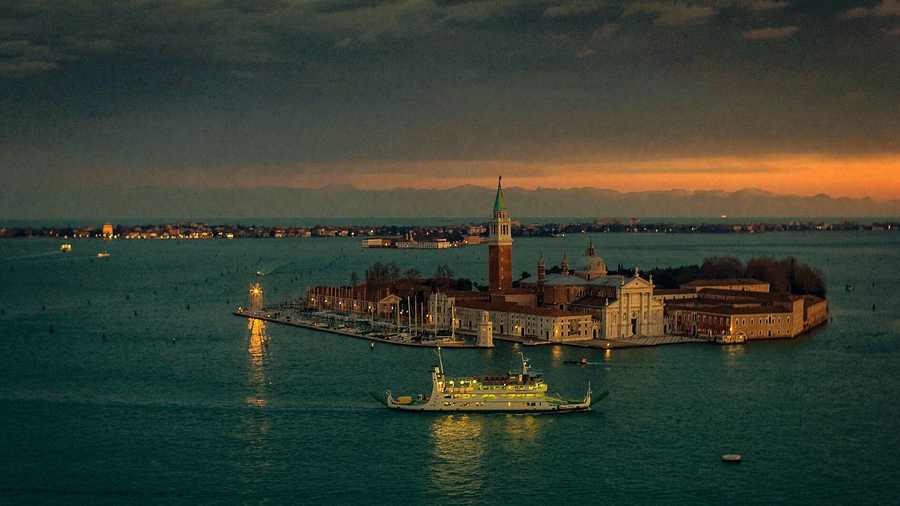</li>
</ul>
</div>
<script src="https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script>
var $carousel = $('.carousel .img-ct').children(),
$btnBefore = $('.btn-before'),
$btnAfter = $('.btn-after'),
$thubms = $('.thubms .sample').children(),
thubmsNumber = $('.thubms .sample').children().length;
var currentKey = 0;
var playing = false;
console.log($carousel);
play(0);
autoPlay();
$btnBefore.on('click', playBefore);
$btnAfter.on('click', playAfter);
$thubms.on('click', function() {
var clickIndex = $(this).index();
play(clickIndex);
})
function autoPlay() {
var beginPlay = setInterval("playAfter()", 3000);
}
function playBefore() {
play((thubmsNumber + currentKey - 1)%thubmsNumber);
}
function playAfter() {
console.log(currentKey+1);
console.log(thubmsNumber);
console.log((currentKey+1)%thubmsNumber);
play((currentKey+1)%thubmsNumber);
console.log(currentKey);
}
function play(index) {
// if(playing) return;
console.log(index);
if(!playing) {
playing = true;
$carousel.eq(currentKey).fadeOut(400);
$carousel.eq(index).fadeIn(400, function() {
playing = false; // 动画异步
});
currentKey = index;
setSample(index, currentKey);
}
}
function setSample(currentKey) {
console.log(currentKey);
$thubms.removeClass('selected').eq(currentKey).addClass('selected');
}
</script>
</body>
</html>
网友评论