本文章翻译于:Material ProgressBar
效果地址
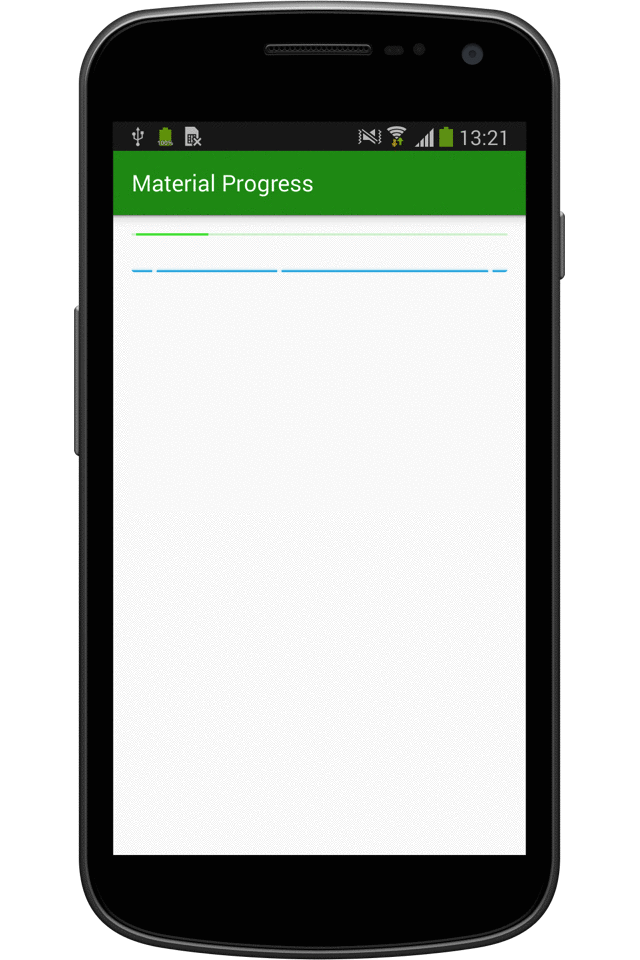
- 虽然效果本身是很容易实现的,问题在于不确定动画看起来相当复杂。而且一个进度条从左到右,但在它的行进的过程中,这个进度条长度是不同的。
- 首先我想到的方法是运用Material来实现,然而这很困难,因为它使用一个AnimatedVectorDrawable这是不可用的。我想出的办法是很狡猾的,但非常接近我们要实现的效果。
- 该方案可以创造我们自己的ProgressBar,完全绕过标准的不确定的逻辑和实现自身的进度和进行的二次行为的ProgressBar。技巧是因为这是如何呈现的,首先是背景,然后是次要的进度,然后是主要的进度。如果我们有背景和基本的颜色相同的颜色,和二次的进展有不同的颜色,我们可以给一个错觉,一个段段的进行绘制。
-
显示这个例子,我们将背景颜色设置为浅绿色,二次进度的颜色为绿色和正在进行的进度的颜色为深绿色,this:
图1
-
然而,如果我们设置原色的次级进度,进行的部分来相匹配的背景色赋予给门绘制的线条的错觉
图2
- 我们可以设置开始跟结束位置,分别设置二级进度条跟总进度条的值。
让我们看下实现代码:
public class MaterialProgressBar extends ProgressBar {
private static final int INDETERMINATE_MAX = 1000;
private static final String SECONDARY_PROGRESS = "secondaryProgress";
private static final String PROGRESS = "progress";
private Animator animator = null;
private final int duration;
public MaterialProgressBar(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
TypedArray ta = context.obtainStyledAttributes(attrs, R.styleable.MaterialProgressBar, defStyleAttr, 0);
int backgroundColour;
int progressColour;
try {
backgroundColour = ta.getColor(R.styleable.MaterialProgressBar_backgroundColour, 0);
progressColour = ta.getColor(R.styleable.MaterialProgressBar_progressColour, 0);
int defaultDuration = context.getResources().getInteger(android.R.integer.config_mediumAnimTime);
duration = ta.getInteger(R.styleable.MaterialProgressBar_duration, defaultDuration);
} finally {
ta.recycle();
}
Resources resources = context.getResources();
setProgressDrawable(resources.getDrawable(android.R.drawable.progress_horizontal));
createIndeterminateProgressDrawable(backgroundColour, progressColour);
setMax(INDETERMINATE_MAX);
super.setIndeterminate(false);
this.setIndeterminate(true);
}
private void createIndeterminateProgressDrawable(@ColorInt int backgroundColour, @ColorInt int progressColour) {
LayerDrawable layerDrawable = (LayerDrawable) getProgressDrawable();
if (layerDrawable != null) {
layerDrawable.mutate();
layerDrawable.setDrawableByLayerId(android.R.id.background, createShapeDrawable(backgroundColour));
layerDrawable.setDrawableByLayerId(android.R.id.progress, createClipDrawable(backgroundColour));
layerDrawable.setDrawableByLayerId(android.R.id.secondaryProgress, createClipDrawable(progressColour));
}
}
private Drawable createClipDrawable(@ColorInt int colour) {
ShapeDrawable shapeDrawable = createShapeDrawable(colour);
return new ClipDrawable(shapeDrawable, Gravity.START, ClipDrawable.HORIZONTAL);
}
private ShapeDrawable createShapeDrawable(@ColorInt int colour) {
ShapeDrawable shapeDrawable = new ShapeDrawable();
setColour(shapeDrawable, colour);
return shapeDrawable;
}
private void setColour(ShapeDrawable drawable, int colour) {
Paint paint = drawable.getPaint();
paint.setColor(colour);
}
.
.
.
}
createIndeterminateProgressDrawable()这个方法用来动态替换LayerDrawable的颜色
现在,我们怎么去动画呢?这一点是非常容易 - 设置我们动画的进度和二次进度值,使用不同的插值,让该段长度在改变动画进度中改变每一段:
public class MaterialProgressBar extends ProgressBar {
.
.
.
@Override
public synchronized void setIndeterminate(boolean indeterminate) {
if (isStarted()) {
return;
}
animator = createIndeterminateAnimator();
animator.setTarget(this);
animator.start();
}
private boolean isStarted() {
return animator != null && animator.isStarted();
}
private Animator createIndeterminateAnimator() {
AnimatorSet set = new AnimatorSet();
Animator progressAnimator = getAnimator(SECONDARY_PROGRESS, new DecelerateInterpolator());
Animator secondaryProgressAnimator = getAnimator(PROGRESS, new AccelerateInterpolator());
set.playTogether(progressAnimator, secondaryProgressAnimator);
set.setDuration(duration);
return set;
}
@NonNull
private ObjectAnimator getAnimator(String propertyName, Interpolator interpolator) {
ObjectAnimator progressAnimator = ObjectAnimator.ofInt(this, propertyName, 0, INDETERMINATE_MAX);
progressAnimator.setInterpolator(interpolator);
progressAnimator.setDuration(duration);
progressAnimator.setRepeatMode(ValueAnimator.RESTART);
progressAnimator.setRepeatCount(ValueAnimator.INFINITE);
return progressAnimator;
}
}
网友评论